Samples Docker labs beginner¶
See also
Contents
docker run hello-world¶
Y:\projects_id3\P5N001\XLOGCA135_tutorial_docker\tutorial_docker>docker run hello-world
Hello from Docker!
This message shows that your installation appears to be working correctly.
To generate this message, Docker took the following steps:
1. The Docker client contacted the Docker daemon.
2. The Docker daemon pulled the "hello-world" image from the Docker Hub.
(amd64)
3. The Docker daemon created a new container from that image which runs the
executable that produces the output you are currently reading.
4. The Docker daemon streamed that output to the Docker client, which sent it
to your terminal.
To try something more ambitious, you can run an Ubuntu container with:
$ docker run -it ubuntu bash
Share images, automate workflows, and more with a free Docker ID:
https://cloud.docker.com/
For more examples and ideas, visit:
https://docs.docker.com/engine/userguide/
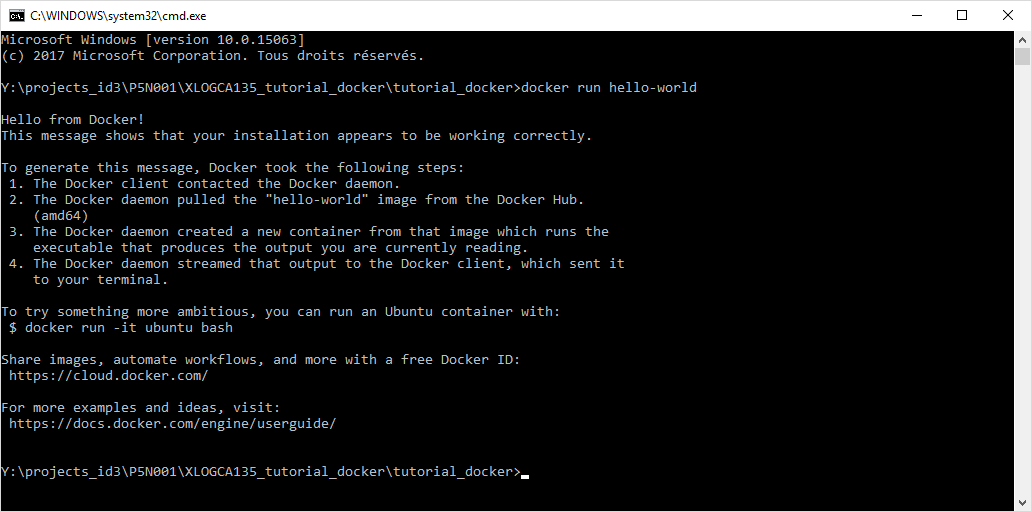
hello.c¶
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 | //#include <unistd.h>
#include <sys/syscall.h>
#ifndef DOCKER_IMAGE
#define DOCKER_IMAGE "hello-world"
#endif
#ifndef DOCKER_GREETING
#define DOCKER_GREETING "Hello from Docker!"
#endif
#ifndef DOCKER_ARCH
#define DOCKER_ARCH "amd64"
#endif
const char message[] =
"\n"
DOCKER_GREETING "\n"
"This message shows that your installation appears to be working correctly.\n"
"\n"
"To generate this message, Docker took the following steps:\n"
" 1. The Docker client contacted the Docker daemon.\n"
" 2. The Docker daemon pulled the \"" DOCKER_IMAGE "\" image from the Docker Hub.\n"
" (" DOCKER_ARCH ")\n"
" 3. The Docker daemon created a new container from that image which runs the\n"
" executable that produces the output you are currently reading.\n"
" 4. The Docker daemon streamed that output to the Docker client, which sent it\n"
" to your terminal.\n"
"\n"
"To try something more ambitious, you can run an Ubuntu container with:\n"
" $ docker run -it ubuntu bash\n"
"\n"
"Share images, automate workflows, and more with a free Docker ID:\n"
" https://cloud.docker.com/\n"
"\n"
"For more examples and ideas, visit:\n"
" https://docs.docker.com/engine/userguide/\n"
"\n";
void _start() {
//write(1, message, sizeof(message) - 1);
syscall(SYS_write, 1, message, sizeof(message) - 1);
//_exit(0);
syscall(SYS_exit, 0);
}
|
Dockerfile.build¶
# explicitly use Debian for maximum cross-architecture compatibility
FROM debian:stretch-slim
RUN dpkg --add-architecture i386
RUN apt-get update && apt-get install -y --no-install-recommends \
gcc \
libc6-dev \
make \
\
libc6-dev:i386 \
libgcc-6-dev:i386 \
\
libc6-dev-arm64-cross \
libc6-dev-armel-cross \
libc6-dev-armhf-cross \
libc6-dev-ppc64el-cross \
libc6-dev-s390x-cross \
\
gcc-aarch64-linux-gnu \
gcc-arm-linux-gnueabi \
gcc-arm-linux-gnueabihf \
gcc-powerpc64le-linux-gnu \
gcc-s390x-linux-gnu \
\
file \
&& rm -rf /var/lib/apt/lists/*
WORKDIR /usr/src/hello
COPY . .
RUN set -ex; \
make clean all test \
TARGET_ARCH='amd64' \
CC='x86_64-linux-gnu-gcc' \
STRIP='x86_64-linux-gnu-strip'
RUN set -ex; \
make clean all \
TARGET_ARCH='arm32v5' \
CC='arm-linux-gnueabi-gcc' \
STRIP='arm-linux-gnueabi-strip'
RUN set -ex; \
make clean all \
TARGET_ARCH='arm32v7' \
CC='arm-linux-gnueabihf-gcc' \
STRIP='arm-linux-gnueabihf-strip'
RUN set -ex; \
make clean all \
TARGET_ARCH='arm64v8' \
CC='aarch64-linux-gnu-gcc' \
STRIP='aarch64-linux-gnu-strip'
RUN set -ex; \
make clean all test \
TARGET_ARCH='i386' \
CC='gcc -m32 -L/usr/lib/gcc/i686-linux-gnu/6' \
STRIP='x86_64-linux-gnu-strip'
RUN set -ex; \
make clean all \
TARGET_ARCH='ppc64le' \
CC='powerpc64le-linux-gnu-gcc' \
STRIP='powerpc64le-linux-gnu-strip'
RUN set -ex; \
make clean all \
TARGET_ARCH='s390x' \
CC='s390x-linux-gnu-gcc' \
STRIP='s390x-linux-gnu-strip'
RUN find \( -name 'hello' -or -name 'hello.txt' \) -exec file '{}' + -exec ls -lh '{}' +
CMD ["./amd64/hello-world/hello"]
Running your first container : docker pull alpine¶
docker pull alpine¶
docker pull alpine
docker images¶
Y:\projects_id3\P5N001\XLOGCA135_tutorial_docker\tutorial_docker>docker images
REPOSITORY TAG IMAGE ID CREATED SIZE
id3pvergain/get-started part2 ed5b70620e49 25 hours ago 148MB
friendlyhello latest ed5b70620e49 25 hours ago 148MB
alpine latest 3fd9065eaf02 6 days ago 4.15MB
wordpress latest 28084cde273b 7 days ago 408MB
centos latest ff426288ea90 7 days ago 207MB
nginx latest 3f8a4339aadd 2 weeks ago 108MB
ubuntu latest 00fd29ccc6f1 4 weeks ago 111MB
python 2.7-slim 4fd30fc83117 5 weeks ago 138MB
hello-world latest f2a91732366c 8 weeks ago 1.85kB
docker4w/nsenter-dockerd latest cae870735e91 2 months ago 187kB
docker run alpine ls -l¶
Y:\projects_id3\P5N001\XLOGCA135_tutorial_docker\tutorial_docker>docker run alpine ls -l
total 52
drwxr-xr-x 2 root root 4096 Jan 9 19:37 bin
drwxr-xr-x 5 root root 340 Jan 16 08:57 dev
drwxr-xr-x 1 root root 4096 Jan 16 08:57 etc
drwxr-xr-x 2 root root 4096 Jan 9 19:37 home
drwxr-xr-x 5 root root 4096 Jan 9 19:37 lib
drwxr-xr-x 5 root root 4096 Jan 9 19:37 media
drwxr-xr-x 2 root root 4096 Jan 9 19:37 mnt
dr-xr-xr-x 127 root root 0 Jan 16 08:57 proc
drwx------ 2 root root 4096 Jan 9 19:37 root
drwxr-xr-x 2 root root 4096 Jan 9 19:37 run
drwxr-xr-x 2 root root 4096 Jan 9 19:37 sbin
drwxr-xr-x 2 root root 4096 Jan 9 19:37 srv
dr-xr-xr-x 13 root root 0 Jan 15 15:33 sys
drwxrwxrwt 2 root root 4096 Jan 9 19:37 tmp
drwxr-xr-x 7 root root 4096 Jan 9 19:37 usr
drwxr-xr-x 11 root root 4096 Jan 9 19:37 var
What happened? Behind the scenes, a lot of stuff happened. When you call run:
- The Docker client contacts the Docker daemon
- The Docker daemon checks local store if the image (alpine in this case) is available locally, and if not, downloads it from Docker Store. (Since we have issued docker pull alpine before, the download step is not necessary)
- The Docker daemon creates the container and then runs a command in that container.
- The Docker daemon streams the output of the command to the Docker client
When you run docker run alpine, you provided a command (ls -l), so Docker started the command specified and you saw the listing.
docker ps -a¶
Liste des conteneurs qui ont tourné à un moment donné.
C:\Tmp>docker ps -a
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
cb62ace67ba4 alpine "ls -l" 20 minutes ago Exited (0) 20 minutes ago eager_heisenberg
685915373a4c hello-world "/hello" 2 hours ago Exited (0) 2 hours ago gallant_wright
e150d0531321 alpine "/bin/sh" 18 hours ago Exited (0) 18 hours ago objective_curran
7d6e93a39de5 alpine "/bin/sh" 18 hours ago Exited (0) 18 hours ago amazing_knuth
807d38ada261 ubuntu "/bin/bash" 18 hours ago Exited (127) 18 hours ago confident_bassi
eebf7e801b96 ubuntu "/bin/bash" 18 hours ago Exited (0) 13 minutes ago wonderful_blackwell
c31e71b41bdb id3pvergain/get-started:part2 "python app.py" 22 hours ago Exited (137) 20 hours ago getstartedlab_web.3.kv05oigiytufm5wsuvnp4guoj
8780b68999cf id3pvergain/get-started:part2 "python app.py" 22 hours ago Exited (137) 20 hours ago getstartedlab_web.4.as0f73cwv5l8fibwnjd60yfyw
f45453da50cf id3pvergain/get-started:part2 "python app.py" 23 hours ago Exited (137) 20 hours ago youthful_wilson
b47fd081642e id3pvergain/get-started:part2 "python app.py" 23 hours ago Exited (137) 20 hours ago admiring_lumiere
06193b763075 friendlyhello "python app.py" 24 hours ago Exited (137) 23 hours ago boring_goodall
16eca9f1274e friendlyhello "python app.py" 26 hours ago Exited (255) 24 hours ago 0.0.0.0:4000->80/tcp stoic_lalande
fb92255412cf hello-world "/hello" 3 days ago Exited (0) 3 days ago infallible_kepler
dd8ca306fb5b hello-world "/hello" 4 days ago Exited (0) 4 days ago musing_hopper
4d1e5f24ba8e nginx "nginx -g 'daemon of…" 4 days ago Exited (0) 4 days ago webserver
docker run -it alpine /bin/sh¶
C:\Tmp>docker run -it alpine /bin/sh
/ # uname -a
Linux 2b8fff5f4068 4.9.60-linuxkit-aufs #1 SMP Mon Nov 6 16:00:12 UTC 2017 x86_64 Linux
/ # ls
bin dev etc home lib media mnt proc root run sbin srv sys tmp usr var
Running the run command with the -it flags attaches us to an interactive tty in the container. Now you can run as many commands in the container as you want. Take some time to run your favorite commands.
That concludes a whirlwind tour of the docker run command which would most likely be the command you’ll use most often.
It makes sense to spend some time getting comfortable with it.
To find out more about run, use docker run –help to see a list of all flags it supports.
As you proceed further, we’ll see a few more variants of docker run.
docker run –help¶
Usage: docker run [OPTIONS] IMAGE [COMMAND] [ARG...]
Run a command in a new container
Options:
--add-host list Add a custom host-to-IP mapping
(host:ip)
-a, --attach list Attach to STDIN, STDOUT or STDERR
--blkio-weight uint16 Block IO (relative weight),
between 10 and 1000, or 0 to
disable (default 0)
--blkio-weight-device list Block IO weight (relative device
weight) (default [])
--cap-add list Add Linux capabilities
--cap-drop list Drop Linux capabilities
--cgroup-parent string Optional parent cgroup for the
container
--cidfile string Write the container ID to the file
--cpu-period int Limit CPU CFS (Completely Fair
Scheduler) period
--cpu-quota int Limit CPU CFS (Completely Fair
Scheduler) quota
--cpu-rt-period int Limit CPU real-time period in
microseconds
--cpu-rt-runtime int Limit CPU real-time runtime in
microseconds
-c, --cpu-shares int CPU shares (relative weight)
--cpus decimal Number of CPUs
--cpuset-cpus string CPUs in which to allow execution
(0-3, 0,1)
--cpuset-mems string MEMs in which to allow execution
(0-3, 0,1)
-d, --detach Run container in background and
print container ID
--detach-keys string Override the key sequence for
detaching a container
--device list Add a host device to the container
--device-cgroup-rule list Add a rule to the cgroup allowed
devices list
--device-read-bps list Limit read rate (bytes per second)
from a device (default [])
--device-read-iops list Limit read rate (IO per second)
from a device (default [])
--device-write-bps list Limit write rate (bytes per
second) to a device (default [])
--device-write-iops list Limit write rate (IO per second)
to a device (default [])
--disable-content-trust Skip image verification (default true)
--dns list Set custom DNS servers
--dns-option list Set DNS options
--dns-search list Set custom DNS search domains
--entrypoint string Overwrite the default ENTRYPOINT
of the image
-e, --env list Set environment variables
--env-file list Read in a file of environment variables
--expose list Expose a port or a range of ports
--group-add list Add additional groups to join
--health-cmd string Command to run to check health
--health-interval duration Time between running the check
(ms|s|m|h) (default 0s)
--health-retries int Consecutive failures needed to
report unhealthy
--health-start-period duration Start period for the container to
initialize before starting
health-retries countdown
(ms|s|m|h) (default 0s)
--health-timeout duration Maximum time to allow one check to
run (ms|s|m|h) (default 0s)
--help Print usage
-h, --hostname string Container host name
--init Run an init inside the container
that forwards signals and reaps
processes
-i, --interactive Keep STDIN open even if not attached
--ip string IPv4 address (e.g., 172.30.100.104)
--ip6 string IPv6 address (e.g., 2001:db8::33)
--ipc string IPC mode to use
--isolation string Container isolation technology
--kernel-memory bytes Kernel memory limit
-l, --label list Set meta data on a container
--label-file list Read in a line delimited file of labels
--link list Add link to another container
--link-local-ip list Container IPv4/IPv6 link-local
addresses
--log-driver string Logging driver for the container
--log-opt list Log driver options
--mac-address string Container MAC address (e.g.,
92:d0:c6:0a:29:33)
-m, --memory bytes Memory limit
--memory-reservation bytes Memory soft limit
--memory-swap bytes Swap limit equal to memory plus
swap: '-1' to enable unlimited swap
--memory-swappiness int Tune container memory swappiness
(0 to 100) (default -1)
--mount mount Attach a filesystem mount to the
container
--name string Assign a name to the container
--network string Connect a container to a network
(default "default")
--network-alias list Add network-scoped alias for the
container
--no-healthcheck Disable any container-specified
HEALTHCHECK
--oom-kill-disable Disable OOM Killer
--oom-score-adj int Tune host's OOM preferences (-1000
to 1000)
--pid string PID namespace to use
--pids-limit int Tune container pids limit (set -1
for unlimited)
--platform string Set platform if server is
multi-platform capable
--privileged Give extended privileges to this
container
-p, --publish list Publish a container's port(s) to
the host
-P, --publish-all Publish all exposed ports to
random ports
--read-only Mount the container's root
filesystem as read only
--restart string Restart policy to apply when a
container exits (default "no")
--rm Automatically remove the container
when it exits
--runtime string Runtime to use for this container
--security-opt list Security Options
--shm-size bytes Size of /dev/shm
--sig-proxy Proxy received signals to the
process (default true)
--stop-signal string Signal to stop a container
(default "15")
--stop-timeout int Timeout (in seconds) to stop a
container
--storage-opt list Storage driver options for the
container
--sysctl map Sysctl options (default map[])
--tmpfs list Mount a tmpfs directory
-t, --tty Allocate a pseudo-TTY
--ulimit ulimit Ulimit options (default [])
-u, --user string Username or UID (format:
<name|uid>[:<group|gid>])
--userns string User namespace to use
--uts string UTS namespace to use
-v, --volume list Bind mount a volume
--volume-driver string Optional volume driver for the
container
--volumes-from list Mount volumes from the specified
container(s)
-w, --workdir string Working directory inside the container
docker inspect alpine¶
C:\Tmp>docker inspect alpine
[
{
"Id": "sha256:3fd9065eaf02feaf94d68376da52541925650b81698c53c6824d92ff63f98353",
"RepoTags": [
"alpine:latest"
],
"RepoDigests": [
"alpine@sha256:7df6db5aa61ae9480f52f0b3a06a140ab98d427f86d8d5de0bedab9b8df6b1c0"
],
"Parent": "",
"Comment": "",
"Created": "2018-01-09T21:10:58.579708634Z",
"Container": "30e1a2427aa2325727a092488d304505780501585a6ccf5a6a53c4d83a826101",
"ContainerConfig": {
"Hostname": "30e1a2427aa2",
"Domainname": "",
"User": "",
"AttachStdin": false,
"AttachStdout": false,
"AttachStderr": false,
"Tty": false,
"OpenStdin": false,
"StdinOnce": false,
"Env": [
"PATH=/usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin"
],
"Cmd": [
"/bin/sh",
"-c",
"#(nop) ",
"CMD [\"/bin/sh\"]"
],
"ArgsEscaped": true,
"Image": "sha256:fbef17698ac8605733924d5662f0cbfc0b27a51e83ab7d7a4b8d8a9a9fe0d1c2",
"Volumes": null,
"WorkingDir": "",
"Entrypoint": null,
"OnBuild": null,
"Labels": {}
},
"DockerVersion": "17.06.2-ce",
"Author": "",
"Config": {
"Hostname": "",
"Domainname": "",
"User": "",
"AttachStdin": false,
"AttachStdout": false,
"AttachStderr": false,
"Tty": false,
"OpenStdin": false,
"StdinOnce": false,
"Env": [
"PATH=/usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin"
],
"Cmd": [
"/bin/sh"
],
"ArgsEscaped": true,
"Image": "sha256:fbef17698ac8605733924d5662f0cbfc0b27a51e83ab7d7a4b8d8a9a9fe0d1c2",
"Volumes": null,
"WorkingDir": "",
"Entrypoint": null,
"OnBuild": null,
"Labels": null
},
"Architecture": "amd64",
"Os": "linux",
"Size": 4147781,
"VirtualSize": 4147781,
"GraphDriver": {
"Data": {
"MergedDir": "/var/lib/docker/overlay2/e4af82b9362c03a84a71a8449c41a37c94592f1e5c2ef1d4f43a255b0a4ee2bd/merged",
"UpperDir": "/var/lib/docker/overlay2/e4af82b9362c03a84a71a8449c41a37c94592f1e5c2ef1d4f43a255b0a4ee2bd/diff",
"WorkDir": "/var/lib/docker/overlay2/e4af82b9362c03a84a71a8449c41a37c94592f1e5c2ef1d4f43a255b0a4ee2bd/work"
},
"Name": "overlay2"
},
"RootFS": {
"Type": "layers",
"Layers": [
"sha256:cd7100a72410606589a54b932cabd804a17f9ae5b42a1882bd56d263e02b6215"
]
},
"Metadata": {
"LastTagTime": "0001-01-01T00:00:00Z"
}
}
]
Next Steps: 2.0 Webapps with Docker¶
For the next step in the tutorial, head over to 2.0 Webapps with Docker.